The error message “Could not find or load main class” is a common issue faced by Java developers, especially beginners. It usually occurs when the Java Virtual Machine (JVM) is unable to locate or properly load the specified class containing the main
method. Resolving this error requires a careful examination of your classpath, package structure, and compilation process.
Understanding the Error
This error typically happens due to one of the following reasons:
- The class name specified in the
java
command does not match the compiled class file. - The file structure does not match the package declaration.
- The classpath is not set correctly when executing the command.
- The compilation process did not generate the appropriate class file.
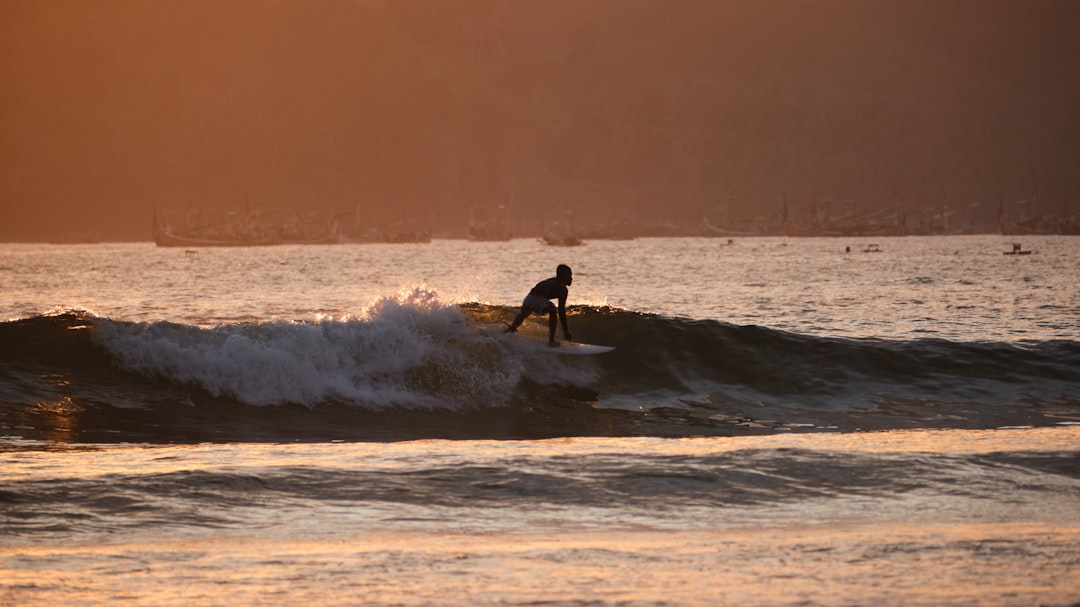
How to Fix the Issue
1. Check the Correct Class Name
One of the most basic mistakes is providing the wrong class name in your execution command. When running a Java program, ensure that you specify the fully qualified name of the class.
For example, if your Java file is named Main.java
, compile and run it as follows:
javac Main.java java Main
If your file is inside a package, such as com.example
, use:
javac com/example/Main.java java com.example.Main
2. Verify the Package and Directory Structure
If your Java file is part of a package, the directory structure must reflect it correctly. Consider the following example:
package com.example; public class Main { public static void main(String[] args) { System.out.println("Hello, World!"); } }
The file should be located inside /com/example/Main.java
. When compiling, ensure you are in the root directory and use:
javac com/example/Main.java java com.example.Main
Running the command from inside the com/example
directory will lead to the “Could not find or load main class” error.
3. Ensure Proper Compilation
Check that your program is actually compiled and that the corresponding .class
file exists. If no class file is found in the expected location, try recompiling the file:
javac Main.java
Then, verify that Main.class
exists in the same directory before running:
java Main
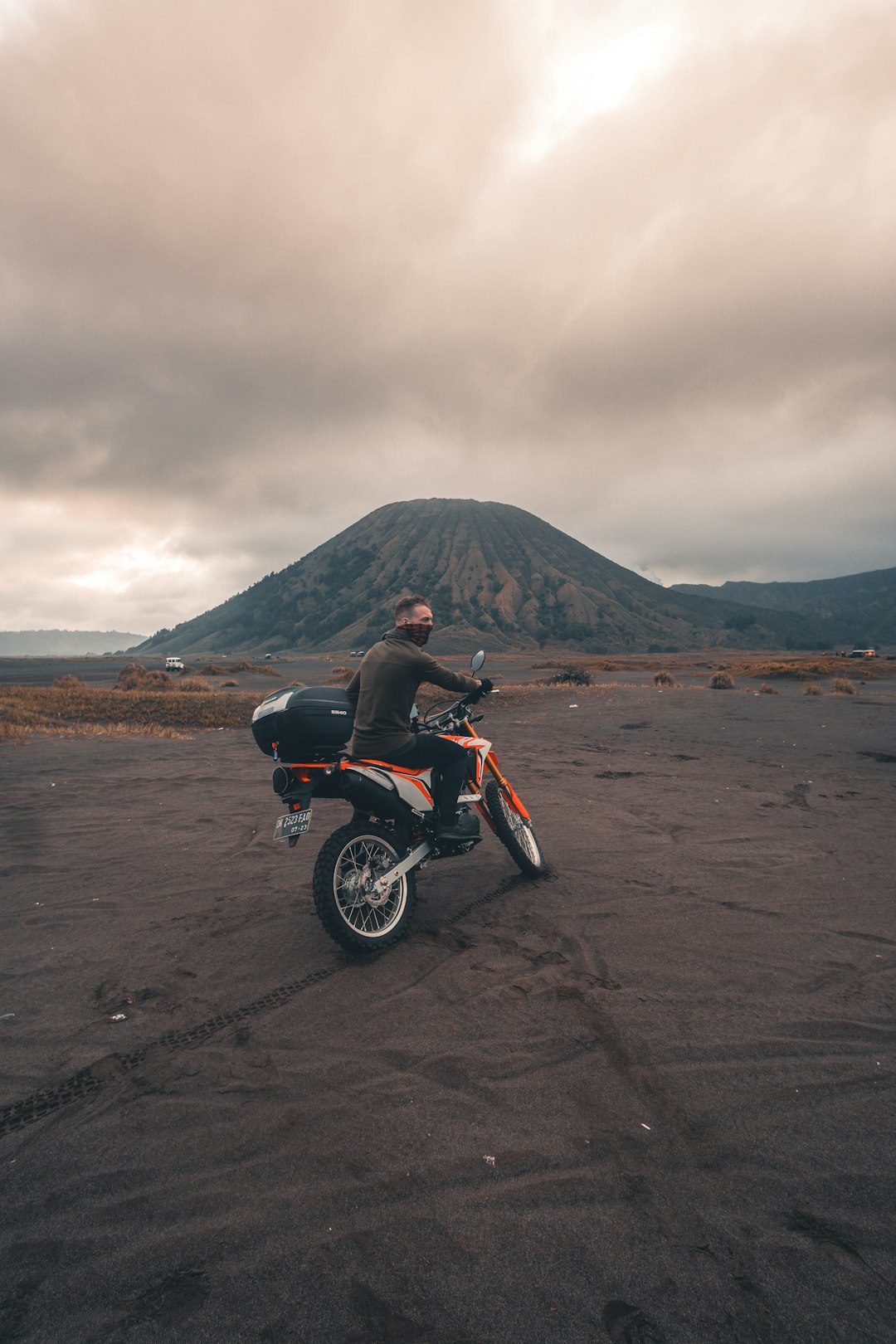
4. Fix Classpath Issues
If your class is in a different location than where you are executing the command, you may need to set the classpath explicitly. The classpath tells Java where to look for compiled class files.
For example, if your class files are stored in /home/user/project/bin
, run:
java -cp /home/user/project/bin Main
For Windows:
java -cp C:\Users\YourName\project\bin Main
5. Avoid Using Extensions in the Command
A common mistake is trying to include the .java
or .class
extension in the execution command. The correct way to run a class is:
java Main
Incorrect formats that cause errors:
java Main.java // Incorrect java Main.class // Incorrect
6. Check for Invisible Characters or Special Formatting
Sometimes, issues arise due to hidden characters or copy-pasting errors in the terminal. If you’re sure your command and class files are correct, try retyping them manually rather than copying and pasting.
Final Thoughts
Understanding and resolving Java errors is a critical skill for software development. The “Could not find or load main class” error often results from incorrect directory structures, classpaths, or naming inconsistencies. By systematically checking these aspects, you can easily diagnose and fix the issue.
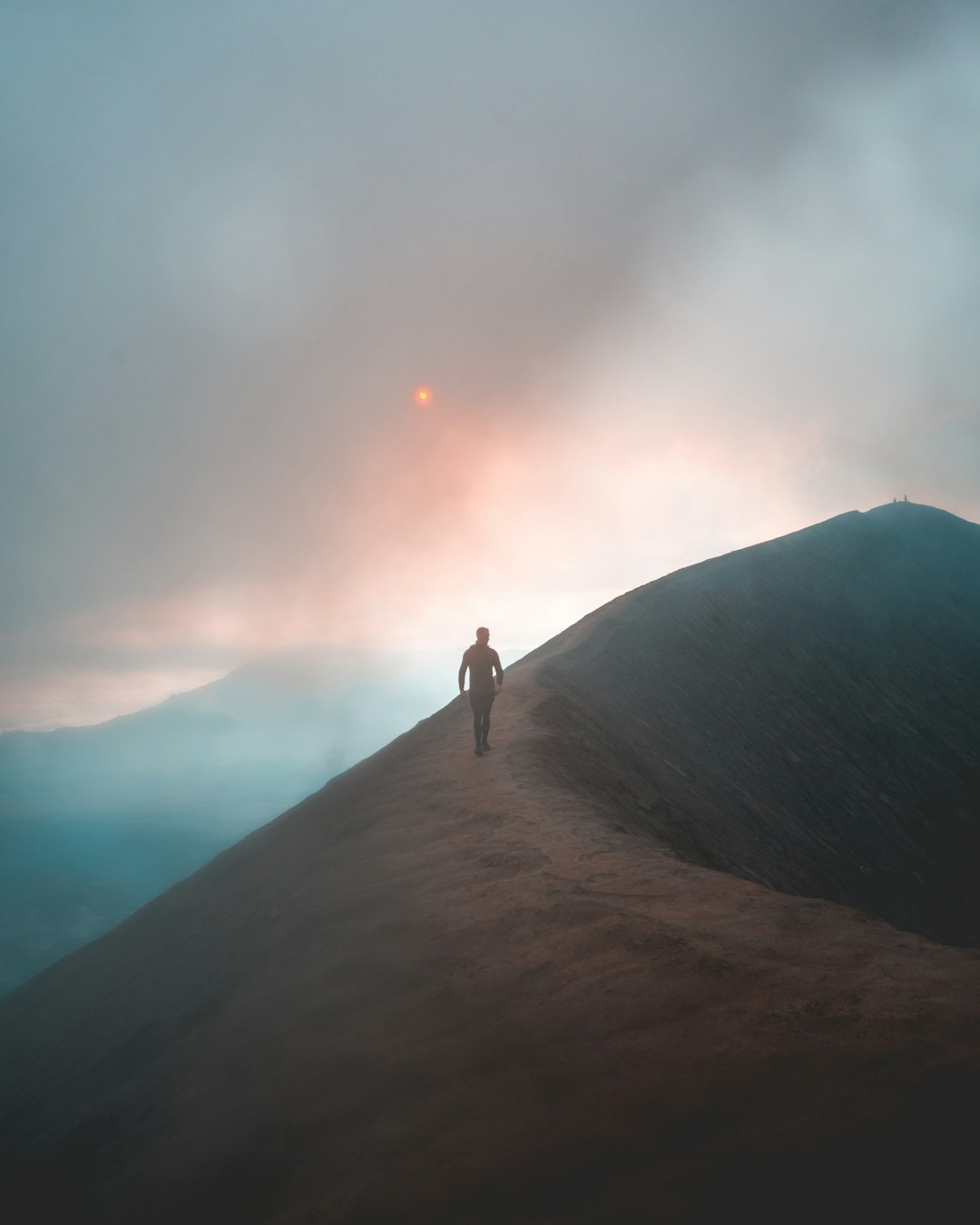
If you’re still facing problems, consider running your program in an IDE such as IntelliJ IDEA or Eclipse, which provides better debugging tools and automated classpath management.
By following these debugging steps, you can ensure a smoother Java development experience and avoid common pitfalls that lead to execution errors.